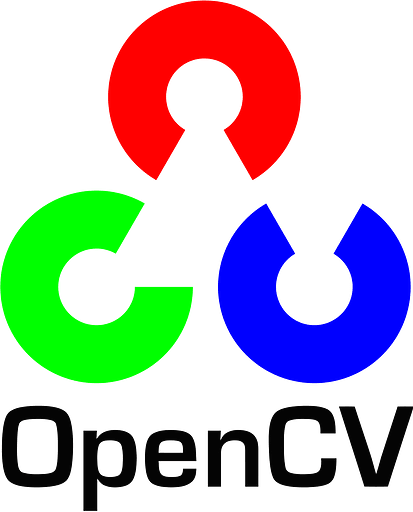
OpenCV Number Plate Recognition
Number plate recognition (NPR) is a type of computer vision application that involves detecting and reading license plates from images or video streams. It has many practical applications, such as traffic enforcement, parking management, toll collection, and more. OpenCV is a powerful tool that can be used for number plate recognition. In this blog post, we will discuss the details of how to implement number plate recognition using OpenCV.
- Preprocessing:
The first step in number plate recognition is to preprocess the input image. This involves converting the image to grayscale, applying filters to remove noise and enhance edges, and segmenting the image into regions of interest (ROIs) that may contain license plates. OpenCV provides a variety of functions for image preprocessing, such as cv2.cvtColor, cv2.GaussianBlur, cv2.Canny, and cv2.findContours.
- Plate Detection:
The next step is to detect candidate plates from the segmented ROIs. This can be done using various techniques such as edge detection, contour analysis, and machine learning algorithms. OpenCV provides a pre-trained Haar cascade classifier that can be used for plate detection. The Haar cascade classifier is a machine learning-based approach that uses a set of positive and negative training images to learn the features of a particular object. Once trained, the classifier can be used to detect the object in new images.
- Plate Recognition:
Once the candidate plates are detected, the next step is to recognize the characters on the plate. This is done using optical character recognition (OCR) techniques. OpenCV provides a variety of OCR algorithms, such as Tesseract OCR, that can be used for this purpose. OCR algorithms work by segmenting the characters on the plate, and then recognizing them based on their shape and features.
- Post-processing:
Finally, the recognized characters are post-processed to eliminate errors and improve accuracy. This may involve applying filters to remove noise, performing character segmentation and recognition again, and validating the recognized characters against a database of known license plates.
here’s an example Python program for number plate recognition using OpenCV:
import cv2
import pytesseract
# Load image
img = cv2.imread('car.jpg')
# Convert to grayscale
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# Apply thresholding
thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)[1]
# Find contours
contours, _ = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# Iterate through contours and find candidate plates
for contour in contours:
x, y, w, h = cv2.boundingRect(contour)
aspect_ratio = w / h
if aspect_ratio > 1.5 and aspect_ratio < 3.5:
plate_img = gray[y:y+h, x:x+w]
# Apply OCR to recognize characters
plate_text = pytesseract.image_to_string(plate_img, config='--psm 11')
# Print recognized characters
print('Number plate:', plate_text)
# Draw bounding box around plate
cv2.rectangle(img, (x, y), (x+w, y+h), (0, 255, 0), 2)
# Display image with bounding boxes and recognized characters
cv2.imshow('Number Plate Recognition', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
This program reads an image of a car and performs number plate recognition using OpenCV and Tesseract OCR. It first converts the image to grayscale, applies thresholding to remove noise, and finds contours. It then iterates through the contours and finds candidate plates by checking their aspect ratio. For each candidate plate, it extracts the plate image and applies OCR to recognize the characters. Finally, it prints the recognized characters and draws a bounding box around the plate on the original image. The result is displayed using OpenCV’s imshow
function.
Conclusion
Number plate recognition using OpenCV is a powerful tool that has many practical applications. By following the steps outlined above, you can implement a basic number plate recognition system using OpenCV. However, it is important to note that number plate recognition can be a complex and challenging task, and there may be many factors that can affect the accuracy of the system, such as lighting conditions, camera angle, and more. Therefore, it is important to carefully evaluate and test the system under various conditions to ensure that it meets your specific needs and requirements.