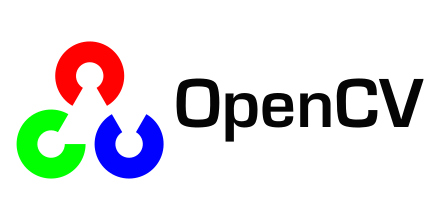
Introduction to OpenCV
OpenCV (Open Source Computer Vision) is an open-source computer vision and machine learning software library that is widely used in a range of applications, including robotics, augmented reality, medical imaging, and computer vision research. OpenCV is typically used in C++, but it also has Python bindings that make it easy to use in Python.
In this blog post, we will provide an overview of using OpenCV in Python, including installation, key features, and sample code.
Installation
OpenCV can be installed in Python using the pip
package manager. Simply open a terminal or command prompt and run the following command:
pip install opencv-python
This will install the latest version of OpenCV for Python.
Key Features
OpenCV provides a wide range of features for image and video processing, including image filtering, feature detection, object recognition, and machine learning. Some of the key features of OpenCV in Python include:
Reading and Writing Images and Videos
OpenCV provides functions for reading and writing images and videos in a range of formats, including JPEG, PNG, and MPEG. These functions make it easy to work with image and video data in Python.
Image Processing
OpenCV provides a wide range of image processing functions in Python, including filtering, thresholding, morphological operations, and color conversion. These functions can be used to manipulate images in a range of ways, from smoothing and sharpening to edge detection and object segmentation.
Object Detection and Tracking
OpenCV provides tools for object detection and tracking in Python, including Haar cascades, feature detection, and template matching. These tools can be used to detect and track objects in images and videos, making it useful for a range of applications, including surveillance and robotics.
Machine Learning
OpenCV provides a range of machine learning algorithms in Python, including k-nearest neighbors, support vector machines, and neural networks. These algorithms can be used for a wide range of applications, including image classification, object detection, and facial recognition.
Sample Code
Here is an example of using OpenCV in Python to read an image from a file, apply a blur filter, and display the result:
import cv2
image = cv2.imread("input.jpg")
blurred_image = cv2.GaussianBlur(image, (3, 3), 0)
cv2.imshow("Output", blurred_image)
cv2.waitKey(0)
In this example, the cv2.imread
function is used to read an image from a file, cv2.GaussianBlur
is used to apply a blur filter to the image, and cv2.imshow
is used to display the result.
OpenCV can be used to build more complex computer vision applications, such as object detection, facial recognition, and augmented reality, making it a powerful tool for developers working in the field of computer vision.